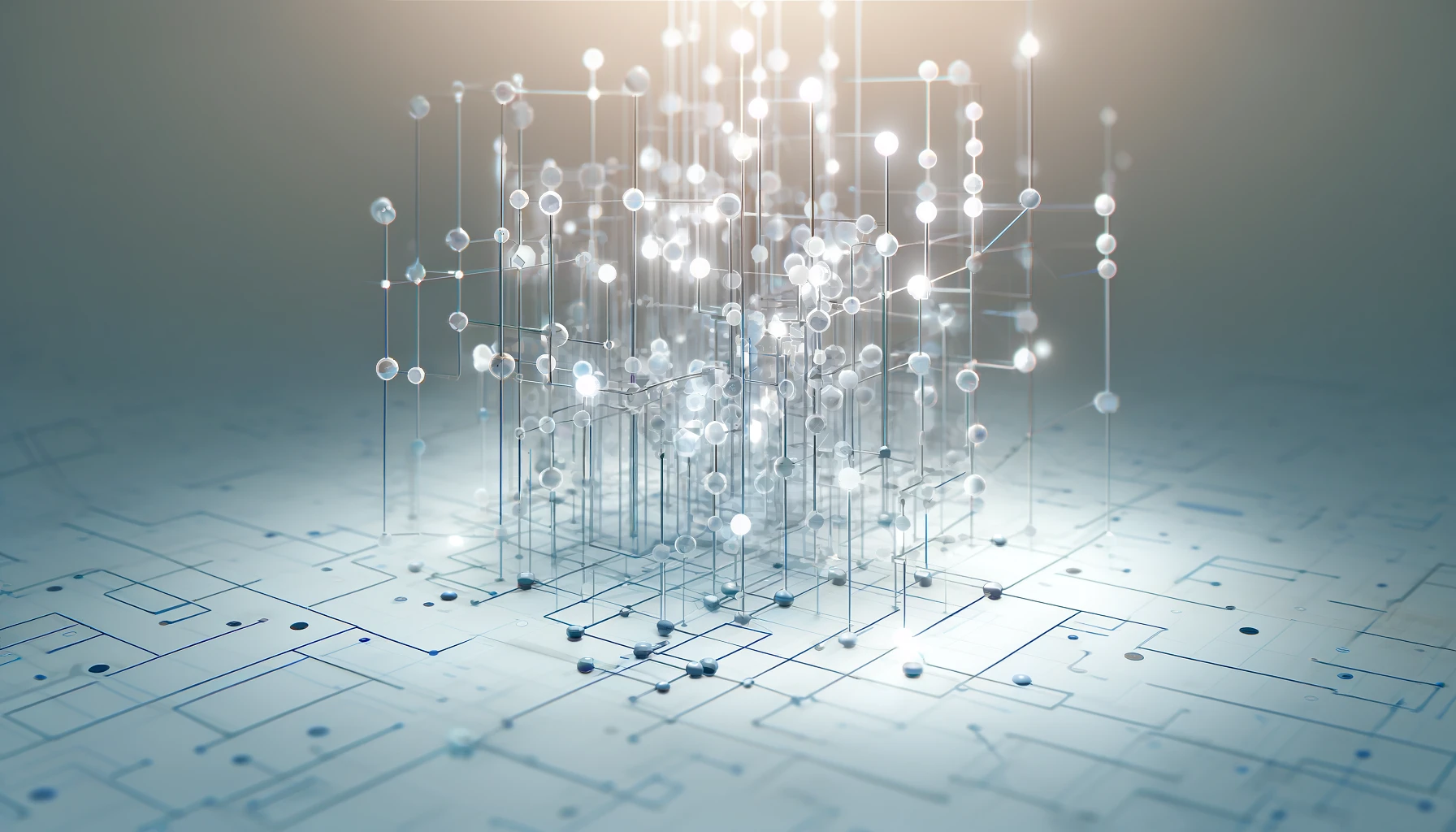
Managing Choice: Enums Over Boolean Flags in Complex Systems
In software development, managing state effectively is crucial for building reliable and maintainable systems. While boolean flags can serve as a simple tool for state management, they often fall short in complex systems where state transitions are numerous and not merely binary. Finite State Machines (FSMs) offer a robust alternative, providing a structured and predictable way to handle state transitions. This blog post explores the advantages of using Finite State Machines over boolean flags, especially in complex environments.
The Limitations of Boolean Flags
Boolean flags are straightforward: they indicate whether a condition is true or false. However, their simplicity can also be a drawback. In complex systems, relying on boolean flags can lead to:
- Increased Complexity: As the number of flags increases, so does the difficulty in managing and understanding their interactions. This can lead to bugs that are hard to trace and fix.
- Hidden Dependencies: Boolean flags often create hidden dependencies and side effects that can make the system unpredictable and difficult to debug.
- Scalability Issues: Scaling a system with numerous boolean flags can become problematic, as adding new states or transitions might require significant changes to existing logic.
Benefits of Finite State Machines
Finite State Machines, on the other hand, offer a more organized and scalable approach to managing state. Here’s why they are better suited for complex systems:
- Clarity and Readability: FSMs provide a clear visual or tabular representation of all states and transitions, making the system easier to understand and maintain.
- Reduced Complexity: By handling the exact state transitions explicitly, FSMs reduce the complexity inherent in managing multiple interdependent flags.
- Predictable Behavior: FSMs make the behavior of a system predictable, as each possible state and transition is mapped out explicitly, avoiding unintended interactions.
- Ease of Debugging: It becomes easier to debug systems using FSMs since you can see the path the system took through its various states up to a point of failure.
Implementing FSMs in Software Systems
To illustrate how FSMs can be implemented in a practical scenario, let’s consider an online order processing system. The states might include “Order Placed,” “Payment Processed,” “Shipped,” and “Delivered.” The transitions between these states are triggered by events like payment confirmation or shipment.
Here’s a simple implementation idea using Python’s transitions
library, which can serve as a conceptual guide:
from transitions import Machine
class Order(object):
states = ['placed', 'paid', 'shipped', 'delivered']
def __init__(self, name):
self.name = name
self.machine = Machine(model=self, states=Order.states, initial='placed')
self.machine.add_transition(trigger='pay', source='placed', dest='paid')
self.machine.add_transition(trigger='ship', source='paid', dest='shipped')
self.machine.add_transition(trigger='deliver', source='shipped', dest='delivered')
order = Order('iPhone')
order.pay() # Transition from 'placed' to 'paid'
order.ship() # Transition from 'paid' to 'shipped'
order.deliver() # Transition from 'shipped' to 'delivered'
FSMs in Larger Contexts
In larger and more complex systems, such as telecommunications networks or manufacturing processes, FSMs become even more essential. They can manage hundreds or even thousands of states and transitions, which would be impractical with boolean flags.
Conclusion
For developers working in complex systems, adopting Finite State Machines is a strategic choice that can lead to better-organized code, easier maintenance, and a more reliable product. While boolean flags have their place in simpler scenarios, FSMs clearly outperform them in handling the nuanced and multi-state processes typical in today’s software applications. Transitioning to FSMs might require a learning curve and initial setup effort, but the long-term benefits in terms of system stability and scalability are well worth it.