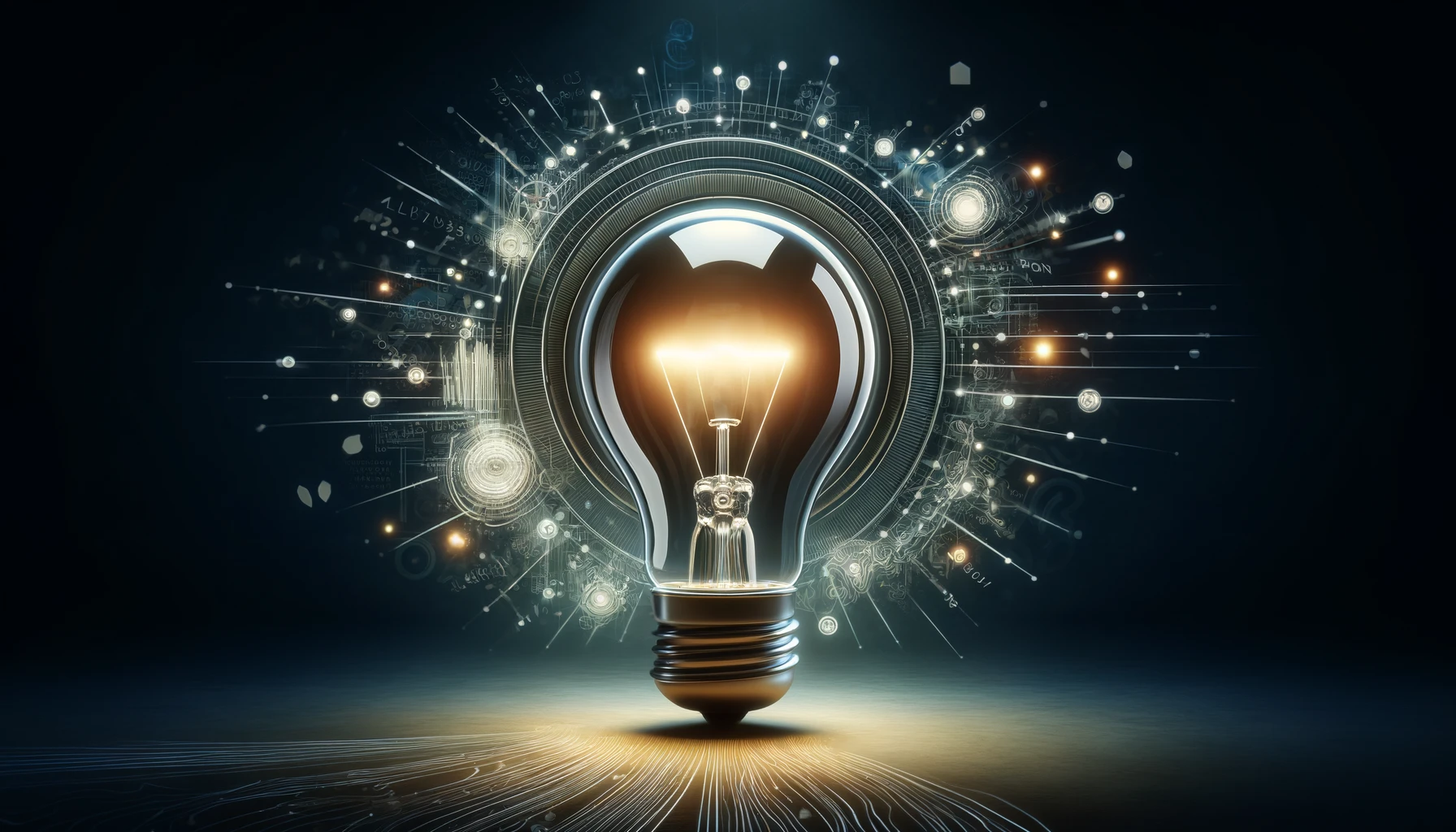
Decoupling Logic: Using The Command Pattern Over Direct Method Calls
In the world of software engineering, the design patterns we choose can drastically influence the flexibility, maintainability, and scalability of our applications. One such pattern, the Command Pattern, offers significant advantages over the more straightforward approach of direct method calls, particularly when it comes to decoupling the components of a system. This post explores why seasoned developers might favor the Command Pattern and how it can lead to cleaner, more robust code.
What is the Command Pattern?
At its core, the Command Pattern encapsulates a request as an object, thereby allowing users to parameterize clients with queues, requests, and operations. It involves four components: the Command, the Receiver, the Invoker, and the Client. The Command interface declares a method for executing a particular action, while the concrete Command classes implement this interface, defining the binding between a Receiver object and an action. The Receiver is the object that knows how to perform the operations associated with carrying out a request. The Invoker holds a command and at some point asks the command to carry out a request. The Client creates a ConcreteCommand object and sets its receiver.
Advantages of the Command Pattern
1. Decoupling the sender and receiver: The foremost benefit of the Command Pattern is the decoupling it achieves between the sender of a request and its receiver. By doing so, it not only simplifies system components but also enhances module independence. This separation allows changes to be made to the implementation details of the receiving object without affecting the clients that issue commands.
2. Extensibility and flexibility: Command classes can be extended and modified independently of the existing client code, promoting flexibility and making it easier to introduce new commands without altering existing code. This feature is particularly beneficial in applications requiring numerous similar operations or actions that trigger complex behaviors.
3. Reversible operations: The pattern can also facilitate operations that require undo capabilities. By maintaining a history of commands, applications can easily rollback to previous states, which is invaluable in software like text editors or graphic design tools that require a robust undo feature.
4. Queue or log requests: Another significant advantage is the ability to queue or log requests. Commands can be serialized into a queue and executed at a later time, a must-have feature in scenarios where actions need to be scheduled, delayed, or repeated independently of the user interface.
5. Integration with other patterns: Often, the Command Pattern is used in conjunction with other patterns such as Composite, which can combine several commands into a single one, further enhancing its utility.
Implementing the Command Pattern
Implementing the Command Pattern might seem overkill for simple applications, but its utility becomes apparent in larger, more complex systems where operations are numerous and involve intricate behaviors or side effects. Here’s a brief snippet to illustrate a simple implementation:
interface Command {
fun execute()
}
class Light {
fun turnOn() = println("The light is on")
fun turnOff() = println("The light is off")
}
class TurnOnCommand(private val light: Light) : Command {
override fun execute() = light.turnOn()
}
class TurnOffCommand(private val light: Light) : Command {
override fun execute() = light.turnOff()
}
class Switch(private val flipOnCommand: Command, private val flipOffCommand: Command) {
fun pressSwitch(isOn: Boolean) {
if (isOn) flipOnCommand.execute() else flipOffCommand.execute()
}
}
In this example, a Light
object’s operation is encapsulated within a FlipUpCommand
, which is triggered by a Switch
. This abstraction allows for changing the behavior of the switch without altering the switch’s code directly, adhering to open/closed principle.
By adopting the Command Pattern, developers can ensure that their software is capable of adapting to change more fluidly, enhancing both functionality and user experience without compromising the system’s integrity.