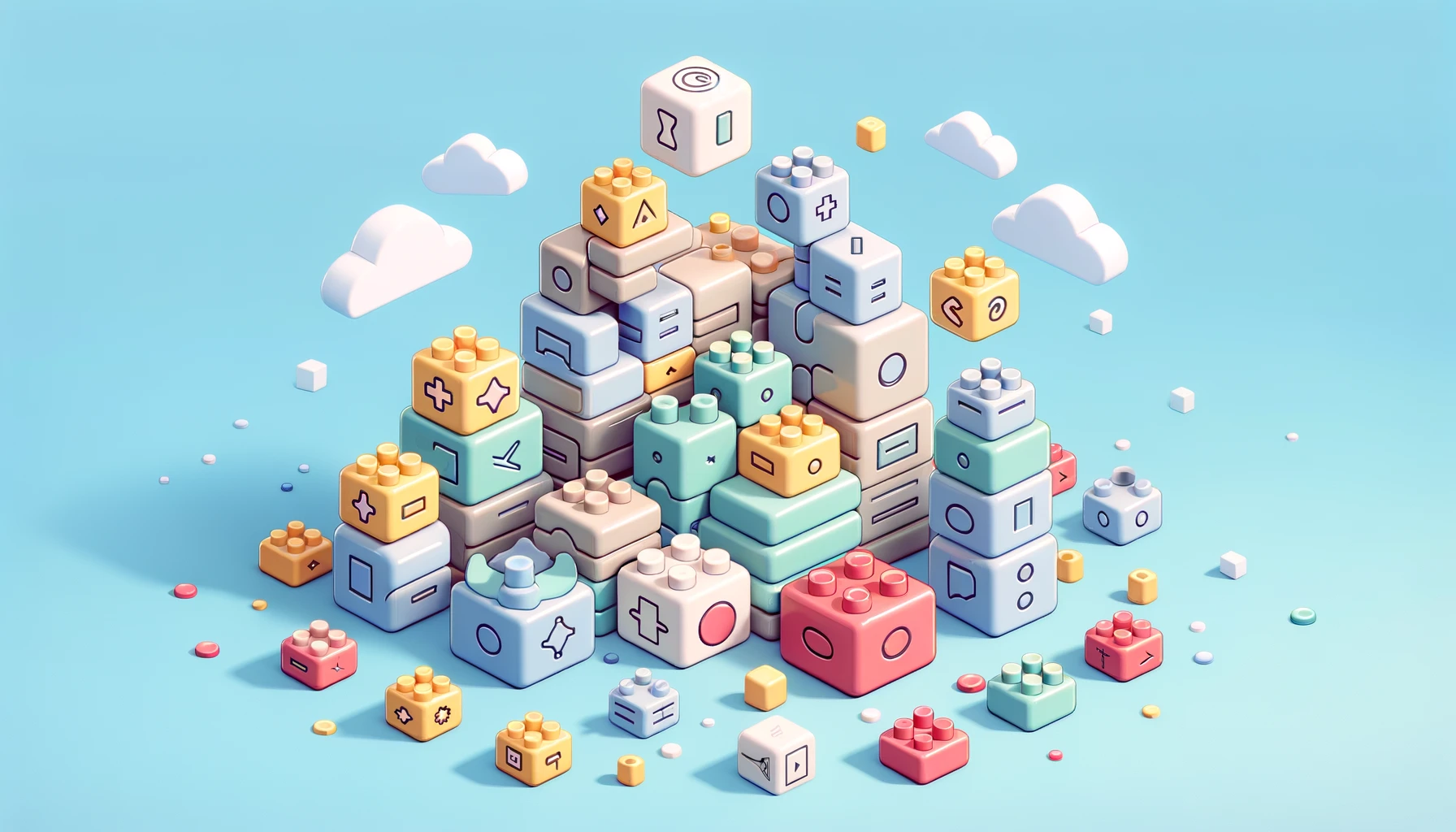
The Hidden Costs of Overusing Inheritance: Composition as a Scalable Alternative
Inheritance is a cornerstone of object-oriented programming, offering a way to promote code reuse and establish a clear hierarchy of classes. However, when used excessively, inheritance can lead to several hidden costs that might compromise the scalability and maintainability of software applications. This blog post explores the drawbacks of overusing inheritance and discusses composition as a more scalable and flexible alternative.
The Pitfalls of Overusing Inheritance
Inheritance allows classes to derive from base classes, inheriting their methods and properties. While this seems beneficial on the surface, overusing inheritance can lead to:
-
Fragile Base Class Problem: Changes to the base class can have unexpected ripple effects on derived classes. If a method is modified in the base class, all derived classes that depend on that method may behave differently, potentially leading to bugs.
-
Tight Coupling: Derived classes are tightly coupled to their base classes, making the system more rigid and less adaptable to change. This coupling complicates the modification of class hierarchies and can lead to a bloated codebase where changes become progressively difficult to manage.
-
Difficulty in Understanding Code: Excessive inheritance can make the codebase difficult to navigate. New developers, or even experienced ones revisiting old code, may find it challenging to trace through layers of inheritance to understand how a particular piece of functionality is implemented.
-
Inheritance Tax: Every subclass inherits not only useful attributes and methods but also those that are irrelevant, which can lead to unnecessary code bloat and reduced performance.
Composition as a Scalable Alternative
Composition involves building classes from other classes by containing instances of other classes in them instead of inheriting from them. This model is often summarized by the principle “favor composition over inheritance.” The advantages of composition include:
-
Increased Flexibility: Composition provides greater flexibility in how functionalities are used and extended in a software application. Objects can acquire properties dynamically at runtime which inheritance typically does not allow.
-
Reduced Coupling: Since objects are designed to delegate responsibilities to other objects, changes in one part of the system are less likely to affect others. This loose coupling makes the system easier to maintain and extend.
-
Enhanced Clarity: With composition, the relationships between different parts of the system are typically easier to understand at a glance. Dependencies are explicit and localized within the composed object, simplifying the mental model of the application’s architecture.
-
Better Control Over Functionality: Composition allows for more control over which behaviors an object exposes since it can select and delegate to specific objects with particular behaviors. This selective use of components avoids the inheritance of unnecessary functionalities.
Implementing Composition in Practice
Consider a simple example where you might traditionally use inheritance. Suppose you have different classes of Vehicle
, such as Car
and Truck
, each inheriting from a Vehicle
class. Using composition, you can create a Vehicle
class that contains objects like Engine
, Wheels
, and Body
, each of which defines its own behaviors and properties. The Vehicle
class can then compose its functionality from these objects, allowing for easy reconfiguration and reuse in different contexts without the constraints imposed by a rigid inheritance hierarchy.
Conclusion
While inheritance has its place in object-oriented programming, relying excessively on it can lead to complications as software grows and evolves. Composition offers a flexible, maintainable, and scalable alternative that can enhance the robustness of your application architecture. By understanding the limitations of inheritance and the benefits of composition, developers can make more informed decisions that lead to cleaner, more efficient codebases.